Track New Signups in Salesforce
You can track new signups in Salesforce with FullContact-Enriched User Profiles, and Send Auth0 Events to MixPanel.
Whenever a new user signs up with a website using a social credential, you want to:
Record a
signup
event in MixPanel.Augment the user profile with additional public information through FullContact.
Record the sign-up as a New Lead in Salesforce, so a sales professional can follow up.
To implement this with Auth0, you need to create three rules in your pipeline. Check out our repository of Auth0 Rules for more great examples.
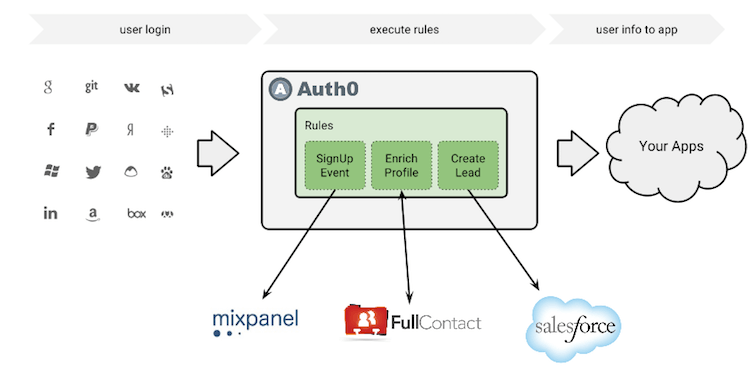
Record sign-up event in MixPanel
Create a rule to record the event by calling MixPanel. In the example below, we record the application name in the application
property to help you filter information in MixPanel. However, the full context
and user
properties are available as sources of additional information (e.g., IP addresses, agent).
This rule will be skipped if the user has already signed up, which is signaled by the user.app_metadata.recordedAsLead
property being set to true
.
function (user, context, callback) {
const request = require('request');
const mpEvent = {
"event": "Sign up",
"properties": {
"distinct_id": user.user_id,
"token": configuration.MIXPANEL_API_TOKEN,
"application": context.clientName
}
};
const base64Event = Buffer.from(JSON.stringify(mpEvent)).toString('base64');
request.get({
url: 'http://api.mixpanel.com/track/',
qs: {
data: base64Event
}
}, (err, res, body) => {
// don’t wait for the MixPanel API call to finish, return right away (the request will continue on the sandbox)`
callback(null, user, context);
});
}
Was this helpful?
Enrich user profile with FullContact
Create a rule to obtain more information about the user by retrieving public information from FullContact's API using the user's email address as input.
Once the call to FullContact completes, we store this additional information in a property called fullContactInfo
.
We ignore certain conditions that exist in the API and only do this when there's a successful call (statusCode=200
). This rule will also be skipped if the user has already signed up, which is signaled by the user.app_metadata.recordedAsLead
property being set to true
.
function (user, context, callback) {
const request = require('request');
const FULLCONTACT_KEY = configuration.FULLCONTACT_KEY;
const SLACK_HOOK = configuration.SLACK_HOOK_URL;
const slack = require('slack-notify')(SLACK_HOOK);
// skip if no email
if (!user.email) return callback(null, user, context);
// skip if fullcontact metadata is already there
if (user.user_metadata && user.user_metadata.fullcontact) return callback(null, user, context);
request.get('https://api.fullcontact.com/v2/person.json', {
qs: {
email: user.email,
apiKey: FULLCONTACT_KEY
},
json: true
}, (error, response, body) => {
if (error || (response && response.statusCode !== 200)) {
slack.alert({
channel: '#slack_channel',
text: 'Fullcontact API Error',
fields: {
error: error ? error.toString() : (response ? response.statusCode + ' ' + body : '')
}
});
// swallow fullcontact api errors and just continue login
return callback(null, user, context);
}
// if we reach here, it means fullcontact returned info and we'll add it to the metadata
user.user_metadata = user.user_metadata || {};
user.user_metadata.fullcontact = body;
auth0.users.updateUserMetadata(user.user_id, user.user_metadata);
context.idToken['https://example.com/fullcontact'] = user.user_metadata.fullcontact;
return callback(null, user, context);
});
}
Was this helpful?