Configure Twilio as MFA SMS Provider
Auth0 has built-in support for sending messages through Twilio. However, you may want to add specific logic before sending a message or send a different message depending on the user or the application. In this case, you would configure SMS MFA to use a Send Phone Message hook.
Twilio provides an SMS messaging service that can be used by Auth0 to deliver multi-factor verification via text messages. It provides two different APIs:
Programmable SMS is a flexible API designed to fully automate SMS communications.
Verify is an API designed to send one-time codes while hides the complexity of SMS delivery.
The following steps will add text-message-based MFA to the login flow for the tenant in which you're working. We highly recommend testing this setup on a staging or development server before making the changes to your production login flow.
To configure a custom SMS provider for MFA using Twilio, you will:
Create a Send Phone Message hook
Configure hook secrets
Add the Twilio call
Add the Twilio Node JS Helper NPM package
Test your hook implementation
Activate the custom SMS factor
Test the MFA flow
Prerequisites
Sign up for a Twilio account.
Create a new Messaging Service in the Programmable SMS console or in the Verify console depending on the API you want to use.
If you use Programmable SMS, you need to add a phone number that is enabled for SMS to your service and capture the number.
If you use the Verify API, you need to make sure that the Twilio Verify Service is configured to accept a custom code. At the time of writing, you need to contact Twilio support to get it enabled.
Capture the Account SID and authorization token by clicking Show API Credentials in the Twilio SMS Dashboard
Create Send Phone Message hook
You will need to create a Send Phone Message hook, which will hold the code and secrets of your custom implementation. You can only have one Send Phone Message hook active at a time.
Configure hook secrets
Add the following hook secrets with the keys and corresponding values:
TWILIO_ACCOUNT_SID
TWILIO_AUTH_TOKEN
TWILIO_PHONE_NUMBER
Add Twilio API call
To make the call to Twilio, add the appropriate code to the hook. Copy one of the code blocks below and edit the Send Phone Message hook code to include it. This function will run each time a user requires MFA, calling Twilio to send a verification code via SMS.
Programmable SMS API
To use the Programmable SMS API, use the code below:
/**
@param {string} recipient - phone number
@param {string} text - message body
@param {object} context - additional authorization context
@param {string} context.factor_type - 'first' or 'second'
@param {string} context.message_type - 'sms' or 'voice'
@param {string} context.action - 'enrollment' or 'authentication'
@param {string} context.language - language used by login flow
@param {string} context.code - one time password
@param {string} context.ip - ip address
@param {string} context.user_agent - user agent making the authentication request
@param {string} context.client_id - to send different messages depending on the client id
@param {string} context.name - to include it in the SMS message
@param {object} context.client_metadata - metadata from client
@param {object} context.user - To customize messages for the user
@param {function} cb - function (error, response)
*/
module.exports = function (recipient, text, context, cb) {
const accountSid = context.webtask.secrets.TWILIO_ACCOUNT_SID;
const authToken = context.webtask.secrets.TWILIO_AUTH_TOKEN;
const fromPhoneNumber = context.webtask.secrets.TWILIO_PHONE_NUMBER;
const client = require("twilio")(accountSid, authToken);
if (context.message_type === "sms") {
client.messages
.create({
body: text,
from: fromPhoneNumber,
to: recipient,
})
.then(function () {
cb(null, {});
})
.catch(function (err) {
cb(err);
});
} else {
const sayInCall = `<?xml version="1.0" encoding="UTF-8"?>
<Response>
<Say voice="man" language="en-US">Hello, your code is {{code}}.</Say>
</Response>`;
client.calls
.create({
twiml: sayInCall,
to: recipient,
from: fromPhoneNumber,
timeout: 30,
})
.then(function () {
cb(null, {});
})
.catch(function (err) {
cb(err);
});
}
};
Was this helpful?
Verify API
To use the Verify API, use the code below:
module.exports = function(recipient, text, context, cb) {
const accountSid = context.webtask.secrets.TWILIO_ACCOUNT_SID;
const authToken = context.webtask.secrets.TWILIO_AUTH_TOKEN;
const verifySid = context.webtask.secrets.TWILIO_VERIFY_SID;
const client = require('twilio')(accountSid, authToken);
client.verify.services(verifySid)
.verifications
.create({
to: recipient,
channel: 'sms',
customCode: context.code
})
.then(function() {
cb(null, {});
})
.catch(function(err) {
cb(err);
});
};
Was this helpful?
Add Twilio Node JS Helper NPM package
The hook uses the Twilio Node.JS Helper Library, so you'll need to include this package in your Hook.
Click the Settings icon and select NPM Modules.
Search for
twilio-node
and add the module that appears.
Test hook implementation
Click the Run icon on the top right to test the hook. Edit the parameters to specify the phone number to receive the SMS, and click the Run button.
Activate custom SMS factor
To use the SMS factor, your tenant needs to have MFA enabled globally or required for specific contexts using rules. To learn how to enable the MFA feature, see:
The hook is now ready to send MFA codes. The last steps are to configure the SMS Factor to use the custom code and test the MFA flow.
Go to Dashboard > Multifactor Auth and click the SMS factor box.
In the modal that appears, select Custom for the SMS Delivery Provider, then make any adjustments you'd like to the templates. Click Save when complete, and close the modal.
Enable the SMS factor using the toggle switch.
Test MFA flow
Trigger an MFA flow and verify that everything works as intended.
Troubleshoot
If you do not receive the text message, look at the hook logs. Look for a failed SMS log entry. To learn which event types to search, see the Log Event Type Code list, or you can use the Filter control to find MFA errors.
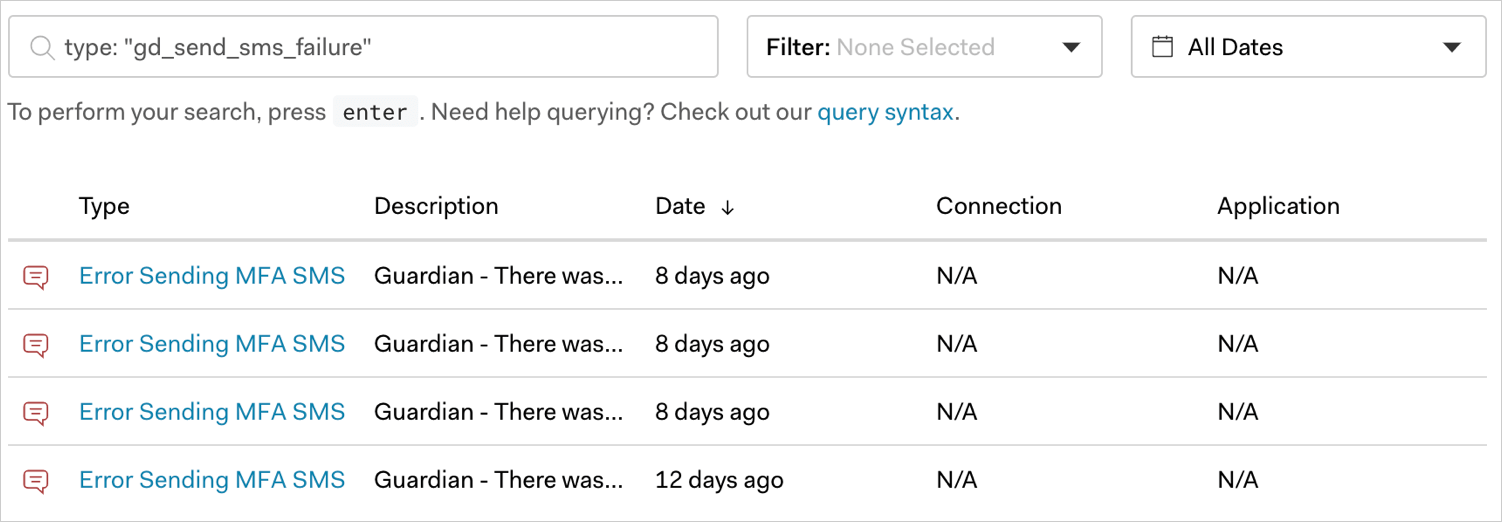
Make sure that:
The Hook is active and the SMS configuration is set to use
Custom
.You have configured the hook secrets.
The configured hook secrets are the same ones you created in the Twilio SMS Dashboard.
You are sending the messages from a phone number that is linked to your Twilio account.
Your phone number is formatted using the E.164 format.